5 Killer Automation Scripts in Python
Here are some awesome automation scripts that you can use in your Python projects.
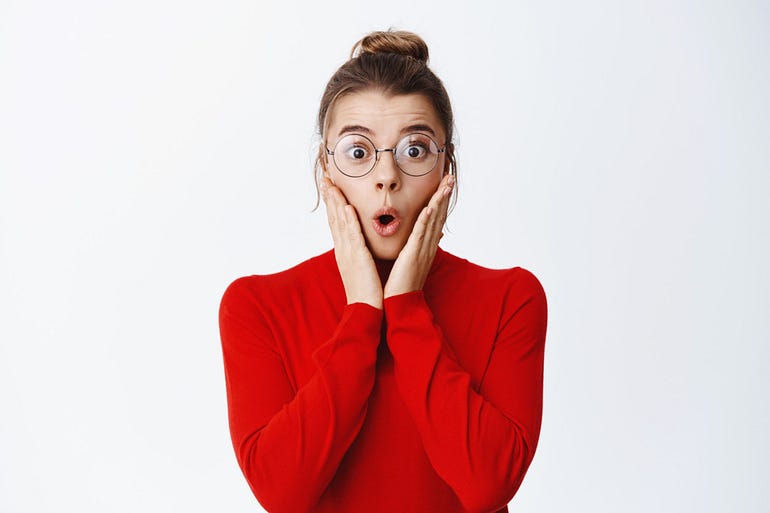
While making projects, we need some ready-made codes that can help us to solve our daily life problems. This article has 5 Automation Scripts for your Python Projects that will solve these problems.
So bookmark it and let’s get started.
1.) Photo Compressor
This will Compress your photos into lower sizes while the quality same.
import PIL
from PIL import Image
from tkinter.filedialog import *fl=askopenfilenames()img = Image.open(fl[0])img.save("result.jpg", "JPEG", optimize = True, quality = 10)
2.) Image Watermarker
This simple script will watermark any image. You can set the Text, location, and Font.
from PIL import Image
from PIL import ImageFont
from PIL import ImageDrawdef watermark_img(img_path,res_path, text, pos):
img = Image.open(img_path)
wm = ImageDraw.Draw(img)
col= (9, 3, 10)
wm.text(pos, text, fill=col)
img.show()
img.save(res_path)img = 'initial.jpg'
watermark_img(img, 'result.jpg','IshaanGupta', pos=(1, 0))
3.) Plagiarism Checker
This script checks Plagiarism between two files.
from difflib import SequenceMatcherdef plagiarism_checker(f1,f2):
with open(f1,errors="ignore") as file1,open(f2,errors="ignore") as file2:
f1_data=file1.read()
f2_data=file2.read()
res=SequenceMatcher(None, f1_data, f2_data).ratio()print(f"These files are {res*100} % similar")f1=input("Enter file_1 path: ")
f2=input("Enter file_2 path: ")
plagiarism_checker(f1, f2)
4.) File Encrypt and Decrypt
A small script that can Encrypt/Decrypt any file.
from cryptography.fernet import Fernetdef encrypt(f_name, key):
fernet = Fernet(key)
with open(f_name, 'rb') as file:
original = file.read()
encrypted = fernet.encrypt(original)
with open(f_name, 'wb') as enc_file:
enc_file.write(encrypted)def decrypt(f_name, key):
fernet = Fernet(key)
with open(f_name, 'rb') as enc_file:
encrypted = enc_file.read()decrypted = fernet.decrypt(encrypted)
with open(f_name, 'wb') as dec_file:
dec_file.write(decrypted)
key = Fernet.generate_key()f_name = input("Enter Your filename: ")
encrypt(f_name, key)
decrypt(f_name, key)
5.) Making URLs short
Sometimes big URLs become very annoying to read and share. This script uses an external API to short the URLs.
from __future__ import with_statement
import contextlib
try:
from urllib.parse import urlencode
except ImportError:
from urllib import urlencodetry:
from urllib.request import urlopen
except ImportError:
from urllib2 import urlopen
import sysdef make_tiny(url):
request_url = ('http://tinyurl.com/api-create.php?' +
urlencode({'url':url}))
with contextlib.closing(urlopen(request_url)) as response:
return response.read().decode('utf-8')def main():
for tinyurl in map(make_tiny, sys.argv[1:]):
print(tinyurl)if __name__ == '__main__':
main()
Final Thoughts
Well, here are some Awesome Automation Python Scripts Part 2 that can be helpful for you to build your projects. I hope you find this article helpful and have learned some new things. Share this article with your Pythoneer Friends.
Comments